Data structure: it is particular way of storing data into computer main memory such that data is stored efficiently both in terms of time and space compelcity.
Simply means that data structure is more efficient when it takes less time and less space to store data.
We want some particular part of data then we can eassly find it.
Data structure os related with computer. But its logical relationship between real world and computer memory

PRIMITIVE DATA STRUCTURE:It’s a inbuilt data type which is already define in compiler.
In primitive data type, you can store only one type of data value. Means that you store only interger or only float or only char or only pointer value.
-
For example:
- interger variable can hold integer type of value
- Float variable can hold floating type of value
- Character variable can hold character type of value
- Pointer variable can hold pointer type
It's a user define data type
In this data type you can create your own data type to store multiple data value.
- Array
- Lists
- Files
Array: An array is a data structure that can hold the elements of same type. It cannot contain the elements of different types like integer with character. The commonly used operation in an array is insertion, deletion, traversing, searching.
For example:
The above example is an array that contains the integer type elements stored in a contiguous manner.
linear data structure is a sequential type of data structure. here sequential means that all the elements in the memory are stored in a sequential manner.for example : element stored after the second element would be the third element, the element stored after the fourth element and so on.
Stack: Stack is a data structure that follows the principle LIFO (Last In First Out). All the operations on the stack are performed from the top of the stack such as PUSH and POP operation. The push operation is the process of inserting element into the stack while the pop operation is the process of removing element from the stack. The stack data structure can be implemented by using either array or linked list.
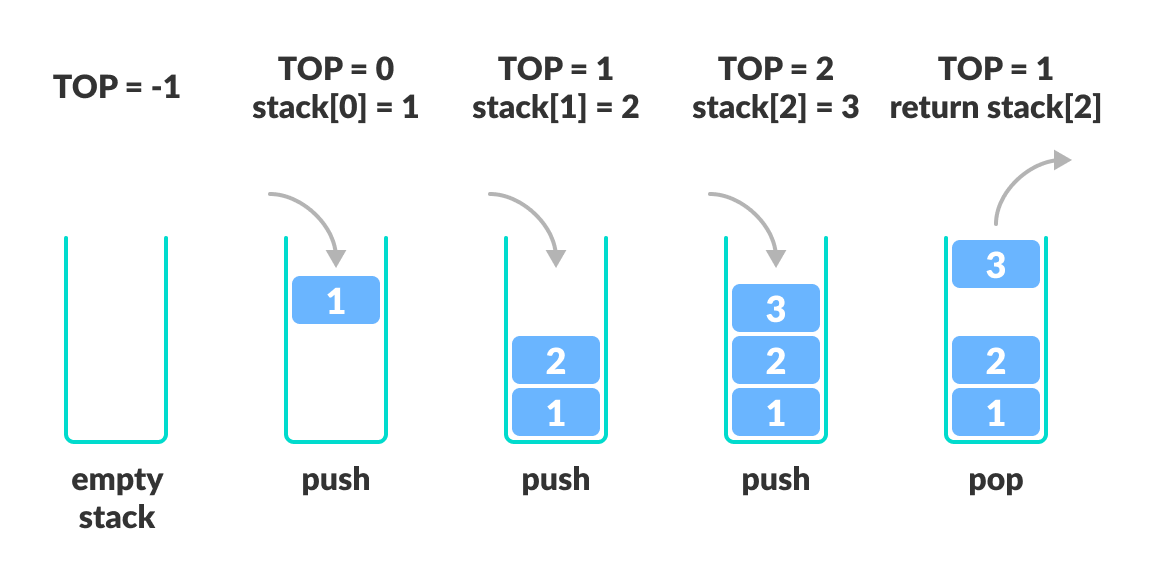
Queue: Queue is a data structure that can be implemented by using array. The difference between the stack and queue data structure is that the elements in the queue are inserted from the rear end while the elements in the queue are removed from the front end.

It's type of data structure, in which the data element are not arranged in a contigous manner.
As the arrangement is non-sequential, so data elements cannot be traversed or accessed in a single run.
If you remember that in linear data structure an element can be connected to more than two elements
Tree : It is a non-linear data structure that consists of various linked nodes. It has a hierarchical tree structure that forms a parent-child relationship. The diagrammatic representation of a tree data structure is shown below:

For example, the posts of employees are arranged in a tree data structure like managers, officers, clerk. In the above figure, A represents a manager, B and C represent the officers, and other nodes represent the clerks.
Graph: It is a non-linear data structure that has a finite number of vertices and edges, and these edges are used to connect the vertices. The vertices are used to store the data elements, while the edges represent the relationship between the vertices. A graph is used in various real-world problems like telephone networks, circuit networks, social networks like LinkedIn, Facebook. In the case of facebook, a single user can be considered as a node, and the connection of a user with others is known as edges.
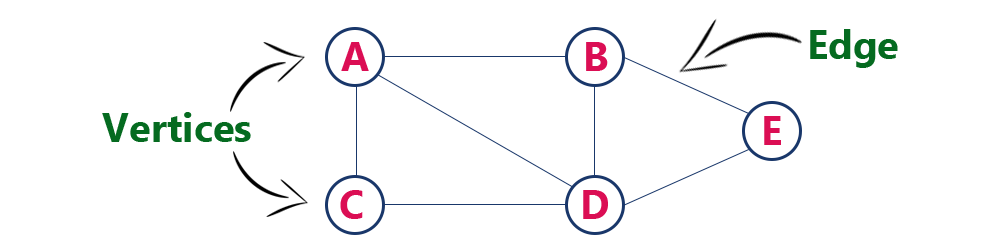
Introduction
Stack is a linear Data Structure in which insertion and deletion of an element can be done from only one end. It is named stack because it has the similar operations as the real-world stacks, for example – pile of books or a pile of plates, etc. Stack uses pointer that always point to the topmost element within the stack, hence called as the top pointer. At any given time we can only access the top element of the stack. The stack follows the LIFO (Last in - First out) structure where the last element inserted would be the first to be deleted. Stack is an Abstract Data Type which can be implemented using array or linked list.
Basic Stack Operations
1.PUSH Operation
When we insert an element in a stack then the operation is known as a push. The element is inserted at the top of the stack. If the stack is full then the overflow condition occurs. When the new element is pushed in a stack, first, the value of the top gets incremented, i.e., top=top+1, and the element will be placed at the new position of the top. The elements can be inserted until we reach the max size of the stack. Let S be name of Stack and maxsize be the max size of the stack. top is the pointer pointing the topmost element starting from 0. data is element to be inserted. **Algorithm for push operation1.[Check is stack is full] if top==maxsize; then write("Overflow Condition") Go to step 4 2.[Increment top] top = top + 1 3.[Insertion of element] S[top] = data 4.[Exit] return
**Code#include stdio.h> int MAXSIZE = 5; int stack[5]; int top = -1; /* Check if the stack is full*/ int isfull(){ if(top == MAXSIZE) return 1; else return 0; } /* Function to insert into the stack */ int push(int data){ if(isfull()) { printf("Stack Overflow \n"); } else { top = top + 1; stack[top] = data; } return 0; } /* Main function */ int main(){ int i; push(44); push(10); push(62); push(62); push(62); push(62); push(62); return 0; }
2.POP Operation
When we delete an element from the stack, the operation is known as a pop. The topmost element is deleted from the stack. If we try to delete the element from the empty stack, then the underflow condition occurs. If the stack is not empty, we first access the element which is pointed by the top. Once the pop operation is performed, the top is decremented by 1, i.e., top=top-1. Let S be name of the stack. top is the pointer pointing the topmost element value be the variable to store the value of deleted element. **Algorithm for pop operation1.[Check is stack is empty] if top== -1; write("Stack Underflow") return 2.[save the value] value = S[top] 3.[decrement the top] top = top - 1 4.[Finished] return value
**Code#include stdio.h int MAXSIZE = 8; int stack[8]; int top = -1; /* Check if the stack is empty */ int isempty(){ if(top == -1) return 1; else return 0; } /* Check if the stack is full*/ int isfull(){ if(top == MAXSIZE) return 1; else return 0; } /* Function to delete from the stack */ int pop(){ int data; if(isempty()) { printf("Stack Underflow"); } else { data = stack[top]; top = top - 1; return data; } } /* Function to insert into the stack */ int push(int data){ if(isfull()) { printf("Could not insert data, Stack is full.\n"); } else { top = top + 1; stack[top] = data; } return 0; } /* Main function */ int main(){ int i; push(44); push(10); push(62); push(123); push(15); int data = pop(); printf("%d ",data); } return 0; }
3.DISPLAY Operation
The display() operation prints all the elements present in the stack. This function is used to display elements of stack to the user. **Algorithm1.[Check if the stack is empty] if top == -1 then write("Stack is empty") return 2.[print the elements of stack] loop for i from 'top' to '0' write("%d ", S[i])
**Code#include stdio.h int MAXSIZE = 8; int stack[8]; int top = -1; /* Check if the stack is full */ int isfull(){ if(top == MAXSIZE) return 1; else return 0; } /* Function to display elements in the stack */ void display(){ if (top == -1){ printf("stack is empty"); return; } for (int i=top; i>=0; i--){ printf("%d ", stack[i]); } } /* Function to insert into the stack */ int push(int data){ if(isfull()) { printf("Could not insert data, Stack is full.\n"); } else { top = top + 1; stack[top] = data; } } /* Main function */ int main(){ int i; push(10); push(44); push(62); display(); return 0; }